Summary
JMenuItem
の内部に切り取り、コピー、貼り付けを行うJButton
を配置します。
Screenshot
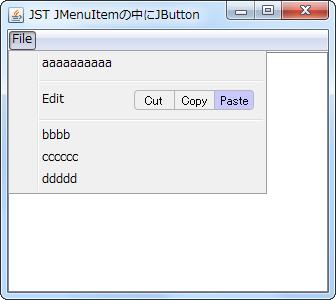
Advertisement
Source Code Examples
private static JMenuItem makeEditMenuItem(final JComponent edit) {
JMenuItem item = new JMenuItem("Edit") {
@Override public Dimension getPreferredSize() {
Dimension d = super.getPreferredSize();
d.width += edit.getPreferredSize().width;
d.height = Math.max(edit.getPreferredSize().height, d.height);
return d;
}
@Override protected void fireStateChanged() {
setForeground(Color.BLACK);
super.fireStateChanged();
}
};
item.setEnabled(false);
GridBagConstraints c = new GridBagConstraints();
item.setLayout(new GridBagLayout());
c.anchor = GridBagConstraints.LINE_END;
c.weightx = 1d;
c.fill = GridBagConstraints.HORIZONTAL;
item.add(Box.createHorizontalGlue(), c);
c.fill = GridBagConstraints.NONE;
item.add(edit, c);
return item;
}
private static JPanel makeEditButtonBar(List<AbstractButton> list) {
int size = list.size();
JPanel p = new JPanel(new GridLayout(1, size, 0, 0)) {
@Override public Dimension getMaximumSize() {
return super.getPreferredSize();
}
};
for (AbstractButton b: list) {
b.setIcon(new ToggleButtonBarCellIcon());
p.add(b);
}
p.setBorder(BorderFactory.createEmptyBorder(4, 10, 4, 10));
p.setOpaque(false);
return p;
}
private static AbstractButton makeButton(String title, Action action) {
JButton b = new JButton(action);
b.addActionListener(new ActionListener() {
@Override public void actionPerformed(ActionEvent e) {
JButton b = (JButton) e.getSource();
Container c = SwingUtilities.getAncestorOfClass(JPopupMenu.class, b);
if (c instanceof JPopupMenu) {
((JPopupMenu) c).setVisible(false);
}
}
});
b.setText(title);
b.setVerticalAlignment(SwingConstants.CENTER);
b.setVerticalTextPosition(SwingConstants.CENTER);
b.setHorizontalAlignment(SwingConstants.CENTER);
b.setHorizontalTextPosition(SwingConstants.CENTER);
b.setBorder(BorderFactory.createEmptyBorder());
b.setContentAreaFilled(false);
b.setFocusPainted(false);
b.setOpaque(false);
b.setBorder(BorderFactory.createEmptyBorder());
return b;
}
View in GitHub: Java, KotlinExplanation
JMenuItem
JMenuItem#getPreferredSize()
をオーバーライドして挿入するJButton
を考慮したサイズを返すように変更JMenuItem
自体は選択不可になるようにJMenuItem#setEnabled(false)
を設定JMenuItem#setEnabled(false)
の状態でも文字色は常に黒になるようにJMenuItem#fireStateChanged
をオーバーライドしてsetForeground(Color.BLACK);
を設定- JRadioButtonの文字色を変更
JMenuItem
にレイアウトを設定しJMenuItem#add(...)
でJButton
を配置したJPanel
を追加- OverlayLayoutの使用
- レイアウトマネージャーは
GridBagLayout
を使用し、追加するJButton
は左右は右端、上下は中央になるよう設定 - GridBagLayoutの使用
JPanel
(JMenuItem
内に右揃えで配置)- レイアウトを
GridLayout
に変更して同じサイズのJButton
を複数配置 JPanel#getMaximumSize()
をオーバーライドしてJPanel#getPreferredSize()
と同じサイズを返すよう変更- JButtonの高さを変更せずに幅を指定
- レイアウトを
JButton
(JPanel
内に3
個等幅で配置)DefaultEditorKit.CopyAction()
などでAction
を設定- 左右両端に配置された
JButton
のフチのラウンドなどはIcon
を生成して描画
Reference
- Custom JMenuItems in Java
- JPopupMenuのレイアウトを変更して上部にメニューボタンを追加する
- こちらは
JPopupMenu
のレイアウトを変更してボタンメニューを作成している
- こちらは
- DefaultEditorKit.CutAction (Java Platform SE 8)
- DefaultEditorKit.CopyAction (Java Platform SE 8)
- DefaultEditorKit.PasteAction (Java Platform SE 8)