JPasswordFieldでパスワードを可視化する
Total: 8689
, Today: 7
, Yesterday: 5
Posted by aterai at
Last-modified:
Summary
JPasswordField
に入力したパスワードの表示・非表示を切り替えるためのボタンを作成し、これを入力欄などに配置します。
Screenshot
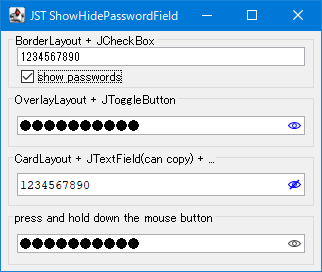
Advertisement
Source Code Examples
JPasswordField pf = new JPasswordField(24);
pf.setText("abcdefghijklmn");
pf.setAlignmentX(Component.RIGHT_ALIGNMENT);
AbstractDocument doc = (AbstractDocument) pf.getDocument();
doc.setDocumentFilter(new ASCIIOnlyDocumentFilter());
AbstractButton b = new JToggleButton(new AbstractAction() {
@Override public void actionPerformed(ActionEvent e) {
AbstractButton c = (AbstractButton) e.getSource();
pf.setEchoChar(c.isSelected()
? '\u0000'
: (Character) UIManager.get("PasswordField.echoChar"));
}
});
b.setFocusable(false);
b.setOpaque(false);
b.setContentAreaFilled(false);
b.setBorder(BorderFactory.createEmptyBorder(0, 0, 0, 4));
b.setAlignmentX(Component.RIGHT_ALIGNMENT);
b.setAlignmentY(Component.CENTER_ALIGNMENT);
b.setIcon(new EyeIcon(Color.BLUE));
b.setRolloverIcon(new EyeIcon(Color.DARK_GRAY));
b.setSelectedIcon(new EyeIcon(Color.BLUE));
b.setRolloverSelectedIcon(new EyeIcon(Color.BLUE));
b.setToolTipText("show/hide passwords");
JPanel panel = new JPanel() {
@Override public boolean isOptimizedDrawingEnabled() {
return false;
}
};
panel.setLayout(new OverlayLayout(panel));
panel.add(b);
panel.add(pf);
View in GitHub: Java, KotlinExplanation
上記のサンプルでは、JPasswordField#setEchoChar(...)
メソッドの値に\u0000
(0
)を設定して入力したパスワードを可視状態に切り替えるためのボタンを追加しています。
BorderLayout + JCheckBox
:BorderLayout
でJPasswordField
の下にパスワード表示非表示切り替え用のJCheckBox
を配置
OverlayLayout + JToggleButton
:OverlayLayout
でJPasswordField
内の右端にパスワード表示非表示切り替え用のJToggleButton
を配置JPasswordField
とJToggleButton
を配置するJPanel
にOverlayLayout
を設定し、マウスカーソルでこのレイアウトのz
軸が入れ替わらないようにJPanel#isOptimizedDrawingEnabled()
が常にfalse
を返すようオーバーライド
CardLayout + JTextField(can copy) + ...
:- 同じ
Document
を使用するJPasswordField
とJTextField
をCardLayout
を設定したJPanel
を作成 OverlayLayout
を設定したJPanel
に上記のJPanel
とJToggleButton
を配置し、JPasswordField
などの内部右端にボタンが表示されるように設定JTextField
をそのまま使用しているので表示中の文字列を選択してコピー可能JPasswordField pf3 = new JPasswordField(24); pf3.setText("abcdefghijklmn"); AbstractDocument doc = (AbstractDocument) pf3.getDocument(); JTextField tf3 = new JTextField(24); tf3.setFont(FONT); tf3.enableInputMethods(false); tf3.setDocument(doc); final CardLayout cardLayout = new CardLayout(); final JPanel p3 = new JPanel(cardLayout); p3.setAlignmentX(Component.RIGHT_ALIGNMENT); p3.add(pf3, PasswordField.HIDE.toString()); p3.add(tf3, PasswordField.SHOW.toString()); AbstractButton b3 = new JToggleButton(new AbstractAction() { @Override public void actionPerformed(ActionEvent e) { AbstractButton c = (AbstractButton) e.getSource(); PasswordField s = c.isSelected() ? PasswordField.SHOW : PasswordField.HIDE; cardLayout.show(p3, s.toString()); } });
- 同じ
press and hold down the mouse button
:OverlayLayout
でJPasswordField
内の右端にパスワード表示非表示切り替え用のJButton
を配置- この
JButton
にMouseListener
を追加してマウスでクリックしている間はパスワードを表示するように設定b4.addMouseListener(new MouseAdapter() { @Override public void mousePressed(MouseEvent e) { pf4.setEchoChar('\u0000'); } @Override public void mouseReleased(MouseEvent e) { pf4.setEchoChar((Character) UIManager.get("PasswordField.echoChar")); } });
passwordField.setEchoChar((char) 0);
を使用するサンプルを追加- JPasswordField#setEchoChar(...) (Java Platform SE 8)に「値
0
に設定すると標準のJTextField
の動作と同様にテキストが入力したとおりに表示されます。」とあるようにpasswordField.setEchoChar((char) 0);
とすればパスワード文字列の表示が可能 CardLayout
を使ってJTextField
を表示する方法は一旦削除したが、表示中のパスワードをコピー可能なので残しておくことにした
- JPasswordField#setEchoChar(...) (Java Platform SE 8)に「値
- ボタンをクリックしている間だけパスワードを表示するサンプルを追加
Reference
- JPasswordField#setEchoChar(...) (Java Platform SE 8)
- JPasswordFieldのエコー文字を変更
- JPasswordFieldでPINコード入力欄を作成する