JPasswordFieldでPINコード入力欄を作成する
Total: 936
, Today: 1
, Yesterday: 0
Posted by aterai at
Last-modified:
Summary
JPasswordField
を使用して数字4
桁のPIN
コード入力欄を作成します。
Screenshot
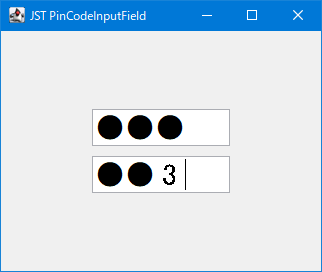
Advertisement
Source Code Examples
class PinCodeDocumentFilter extends DocumentFilter {
public static final int MAX = 4;
@Override public void replace(
DocumentFilter.FilterBypass fb, int offset,
int length, String text, AttributeSet attrs) throws BadLocationException {
String str = fb.getDocument().getText(
0, fb.getDocument().getLength()) + text;
if (str.length() <= MAX && str.matches("\\d+")) {
super.replace(fb, offset, length, text, attrs);
}
}
}
class PasswordView2 extends PasswordView {
public PasswordView2(Element elem) {
super(elem);
}
@Override protected int drawUnselectedText(
Graphics g, int x, int y, int p0, int p1) throws BadLocationException {
return drawText(g, x, y, p0, p1);
}
// @see drawUnselectedTextImpl(...)
private int drawText(Graphics g, int x, int y, int p0, int p1)
throws BadLocationException {
Container c = getContainer();
int j = x;
if (c instanceof JPasswordField) {
JPasswordField f = (JPasswordField) c;
if (f.isEnabled()) {
g.setColor(f.getForeground());
} else {
g.setColor(f.getDisabledTextColor());
}
Graphics2D g2 = (Graphics2D) g;
char echoChar = f.getEchoChar();
int n = p1 - p0;
for (int i = 0; i < n; i++) {
j = i == n - 1
? drawLastChar(g2, j, y, i)
: drawEchoCharacter(g, j, y, echoChar);
}
}
return j;
}
private int drawLastChar(Graphics g, int x, int y, int p1)
throws BadLocationException {
Graphics2D g2 = (Graphics2D) g;
Font font = g2.getFont();
float fs = font.getSize2D();
double w = font.getStringBounds("0", g2.getFontRenderContext()).getWidth();
int sz = (int) ((fs - w) / 2d);
Document doc = getDocument();
Segment s = new Segment(); // SegmentCache.getSharedSegment();
doc.getText(p1, 1, s);
// int ret = Utilities.drawTabbedText(s, x, y, g, this, p1);
// SegmentCache.releaseSharedSegment(s);
return Utilities.drawTabbedText(s, x + sz, y, g, this, p1);
}
}
View in GitHub: Java, KotlinDescription
PinCodeDocumentFilter
をJPasswordField
に設定して4
桁の0-9
数字のみ入力可能になるよう制限JPasswordField#setColumns(4)
で表示桁を4
桁に設定する4
文字目のエコー文字が見切れてしまう?
PlaceholderLayerUI
をJPasswordField
に設定して入力欄が空の場合はヒント文字列を表示するDocumentListener
をJPasswordField
のDocument
に設定して4
桁の数字が入力されたらJOptionPane
の表示と入力文字列のリセットを実行JPasswordField#setText("")
でのリセットはEventQueue.invokeLater(...)
などで後で実行しないとIllegalStateException
が発生する場合がある?
DefaultCaret#isSelectionVisible()
が常にfalse
を返すようオーバライドしたCaret
をJPasswordField
に設定して文字列選択を非表示化BasicPasswordFieldUI#create(Element)
をオーバーライドして末尾の数字のみマスクせずに可視化するPasswordView2
を返すよう設定
JPasswordField password = new JPasswordField(6) {
@Override public void updateUI() {
super.updateUI();
setUI(new BasicPasswordFieldUI() {
@Override public View create(Element elem) {
return new PasswordView2(elem);
}
});
}
};
Reference
- [JDK-8296878] Document Filter attached to JPasswordField and setText("") is not cleared instead inserted characters replaced with unicode null characters - Java Bug System
- ComboBoxEditorにJLayerを設定してプレースホルダ文字列を表示する
- JTextFieldが編集不可状態でもCaretが点滅するよう設定する
- JPasswordFieldでパスワードを可視化する