Summary
円形やラウンド矩形などの任意の図形を適用したJButton
を作成します。
Screenshot
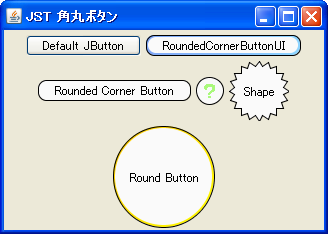
Advertisement
Source Code Examples
class RoundedCornerButton extends JButton {
private static final float ARC_WIDTH = 16f;
private static final float ARC_HEIGHT = 16f;
protected static final int FOCUS_STROKE = 2;
protected final Color fc = new Color(100, 150, 255, 200);
protected final Color ac = new Color(230, 230, 230);
protected final Color rc = Color.ORANGE;
protected Shape shape;
protected Shape border;
protected Shape base;
public RoundedCornerButton() {
super();
}
public RoundedCornerButton(Icon icon) {
super(icon);
}
public RoundedCornerButton(String text) {
super(text);
}
public RoundedCornerButton(Action a) {
super(a);
// setAction(a);
}
public RoundedCornerButton(String text, Icon icon) {
super(text, icon);
// setModel(new DefaultButtonModel());
// init(text, icon);
// setContentAreaFilled(false);
// setBackground(new Color(250, 250, 250));
// initShape();
}
@Override public void updateUI() {
super.updateUI();
setContentAreaFilled(false);
setFocusPainted(false);
setBackground(new Color(250, 250, 250));
initShape();
}
protected void initShape() {
if (!getBounds().equals(base)) {
base = getBounds();
shape = new RoundRectangle2D.Float(
0, 0, getWidth() - 1, getHeight() - 1, ARC_WIDTH, ARC_HEIGHT);
border = new RoundRectangle2D.Float(
FOCUS_STROKE, FOCUS_STROKE, getWidth() - 1 - FOCUS_STROKE * 2,
getHeight() - 1 - FOCUS_STROKE * 2, ARC_WIDTH, ARC_HEIGHT);
}
}
private void paintFocusAndRollover(Graphics2D g2, Color color) {
g2.setPaint(new GradientPaint(
0, 0, color, getWidth() - 1, getHeight() - 1,
color.brighter(), true));
g2.fill(shape);
g2.setColor(getBackground());
g2.fill(border);
}
@Override protected void paintComponent(Graphics g) {
initShape();
Graphics2D g2 = (Graphics2D) g.create();
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
if (getModel().isArmed()) {
g2.setColor(ac);
g2.fill(shape);
} else if (isRolloverEnabled() && getModel().isRollover()) {
paintFocusAndRollover(g2, rc);
} else if (hasFocus()) {
paintFocusAndRollover(g2, fc);
} else {
g2.setColor(getBackground());
g2.fill(shape);
}
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_OFF);
g2.setColor(getBackground());
super.paintComponent(g2);
g2.dispose();
}
@Override protected void paintBorder(Graphics g) {
initShape();
Graphics2D g2 = (Graphics2D) g.create();
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
g2.setColor(getForeground());
g2.draw(shape);
g2.dispose();
}
@Override public boolean contains(int x, int y) {
initShape();
return shape == null ? false : shape.contains(x, y);
}
}
View in GitHub: Java, KotlinExplanation
- 形や縁、クリック可能な領域をラウンド矩形などの
Shape
に変更したボタンを作成JButton#paintComponent()
メソッドをオーバーライドして描画を変更JButton#contains()
メソッドをオーバーライドしてマウスでクリック可能な領域を変更
- 角丸ボタンを継承して円ボタンを作成
- 幅と高さが同じになるように
getPreferredSize()
メソッドをオーバーライド - 図形の初期化メソッドをオーバーライド
- メソッド名前は適当、上記のサンプルでは
initShape()
- メソッド名前は適当、上記のサンプルでは
- 幅と高さが同じになるように
class RoundButton extends RoundedCornerButton {
public RoundButton(String text) {
super(text);
setFocusPainted(false);
initShape();
}
@Override public Dimension getPreferredSize() {
Dimension d = super.getPreferredSize();
d.width = d.height = Math.max(d.width, d.height);
return d;
}
protected void initShape() {
if (!getBounds().equals(base)) {
base = getBounds();
shape = new Ellipse2D.Float(0, 0, getWidth() - 1, getHeight() - 1);
border = new Ellipse2D.Float(focusstroke, focusstroke,
getWidth() - 1 - focusstroke * 2,
getHeight() - 1 - focusstroke * 2);
}
}
}