Fontを回転する
Total: 15142
, Today: 2
, Yesterday: 11
Posted by aterai at
Last-modified:
概要
Font
から文字のアウトラインを取得し、その中心をアンカーポイントに設定して回転します。
Screenshot
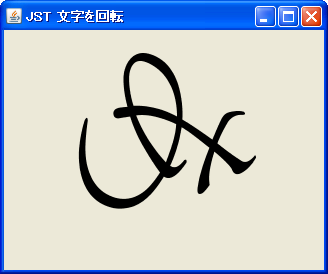
Advertisement
サンプルコード
class FontRotateAnimation extends JComponent implements ActionListener {
private final Timer animator;
private int rotate;
private final Shape shape;
private Shape s;
public FontRotateAnimation(String str) {
super();
animator = new Timer(10, this);
addHierarchyListener(new HierarchyListener() {
@Override public void hierarchyChanged(HierarchyEvent e) {
if ((e.getChangeFlags() & HierarchyEvent.DISPLAYABILITY_CHANGED) != 0
&& animator != null && !e.getComponent().isDisplayable()) {
animator.stop();
}
}
});
Font font = new Font(Font.SERIF, Font.PLAIN, 200);
FontRenderContext frc = new FontRenderContext(null, true, true);
shape = new TextLayout(str, font, frc).getOutline(null);
s = shape;
animator.start();
}
@Override protected void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D) g.create();
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
g2.setPaint(Color.BLACK);
g2.fill(s);
g2.dispose();
}
@Override public void actionPerformed(ActionEvent e) {
repaint(s.getBounds());
Rectangle2D b = shape.getBounds2D();
Point2D p = new Point2D.Double(
b.getX() + b.getWidth() / 2d, b.getY() + b.getHeight() / 2d);
AffineTransform at = AffineTransform.getRotateInstance(
Math.toRadians(rotate), p.getX(), p.getY());
AffineTransform toCenterAT = AffineTransform.getTranslateInstance(
getWidth() / 2d - p.getX(), getHeight() / 2d - p.getY());
Shape s1 = at.createTransformedShape(shape);
s = toCenterAT.createTransformedShape(s1);
repaint(s.getBounds());
rotate = rotate >= 360 ? 0 : rotate + 2;
}
}
View in GitHub: Java, Kotlin解説
上記のサンプルでは、対象文字、Font
、FontRenderContext
からTextLayout
を生成し、TextLayout#getOutline()
メソッドで文字のアウトラインをShape
として取得しています。
OpenJDK Corretto-8.212
で実行すると回転中にフォント表示が乱れる件は、Java2D rendering may break when using soft clipping effects · Issue #127 · corretto/corretto-8、8u222
で修正済みされる予定