TextLayoutでFontのメトリック情報を取得する
Total: 6849
, Today: 2
, Yesterday: 1
Posted by aterai at
Last-modified:
Summary
TextLayout
からFont
のAscent
、Descent
、Leading
などのメトリック情報を取得して描画します。
Screenshot
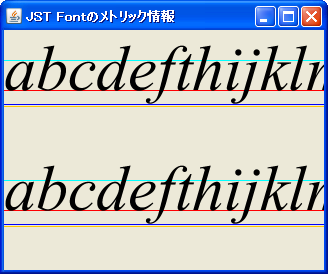
Advertisement
Source Code Examples
String text = "abcdefthijklmnopqrstuvwxyz";
Font font = new Font(Font.SERIF, Font.ITALIC, 64);
FontRenderContext frc = new FontRenderContext(null, true, true);
TextLayout tl = new TextLayout(text, font, frc);
@Override protected void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
int w = getWidth();
float baseline = getHeight() / 2f;
g2.setPaint(Color.RED);
g2.draw(new Line2D.Float(0, baseline, w, baseline));
g2.setPaint(Color.GREEN);
float ascent = baseline - tl.getAscent();
g2.draw(new Line2D.Float(0, ascent, w, ascent));
g2.setPaint(Color.BLUE);
float descent = baseline + tl.getDescent();
g2.draw(new Line2D.Float(0, descent, w, descent));
g2.setPaint(Color.ORANGE);
float leading = baseline + tl.getDescent() + tl.getLeading();
g2.draw(new Line2D.Float(0, leading, w, leading));
g2.setPaint(Color.CYAN);
float xheight = baseline - (float) tl.getBlackBoxBounds(23, 24).getBounds().getHeight();
g2.draw(new Line2D.Float(0, xheight, w, xheight));
g2.setPaint(Color.BLACK);
tl.draw(g2, 0f, baseline);
}
View in GitHub: Java, KotlinExplanation
上記のサンプルでは、上の文字列はTextLayout
を使用して、下はGlyphVector
+ LineMetrics
でFont
のメトリック情報を取得してガイドラインを描画しています。
Color.RED
- ベースライン
Color.GREEN
:Ascent
- ベースライン -
Ascent
- ベースライン -
Color.BLUE
:Descent
- ベースライン +
Descent
- ベースライン +
Color.ORANGE
:Leading
- ベースライン +
Descent
+Leading
- ベースライン +
Color.CYAN
:x-height
- ベースライン - 小文字
x
の高さ
- ベースライン - 小文字