JLabelの文字列を折り返し
Total: 42495
, Today: 2
, Yesterday: 10
Posted by aterai at
Last-modified:
Summary
GlyphVector
を使って、ラベルの文字列を折り返して表示します。
Screenshot
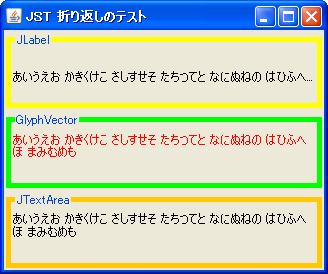
Advertisement
Source Code Examples
class WrappedLabel extends JLabel {
private GlyphVector gvtext;
private int width = -1;
public WrappedLabel() {
this(null);
}
public WrappedLabel(String str) {
super(str);
}
@Override public void doLayout() {
Insets i = getInsets();
int w = getWidth() - i.left - i.right;
if (w != width) {
Font font = getFont();
FontMetrics fm = getFontMetrics(font);
FontRenderContext frc = fm.getFontRenderContext();
gvtext = getWrappedGlyphVector(getText(), w, font, frc);
width = w;
}
super.doLayout();
}
@Override protected void paintComponent(Graphics g) {
if (gvtext == null) {
super.paintComponent(g);
} else {
Insets i = getInsets();
Graphics2D g2 = (Graphics2D) g.create();
g2.setPaint(Color.RED);
g2.drawGlyphVector(gvtext, i.left, getFont().getSize() + i.top);
g2.dispose();
}
}
private GlyphVector getWrappedGlyphVector(
String str, float width, Font font, FontRenderContext frc) {
Point2D gmPos = new Point2D.Double(0d, 0d);
GlyphVector gv = font.createGlyphVector(frc, str);
float lineheight = (float) (gv.getLogicalBounds().getHeight());
float xpos = 0f;
float advance = 0f;
int lineCount = 0;
GlyphMetrics gm;
for (int i = 0; i < gv.getNumGlyphs(); i++) {
gm = gv.getGlyphMetrics(i);
advance = gm.getAdvance();
if (xpos < width && width <= xpos + advance) {
lineCount++;
xpos = 0f;
}
gmPos.setLocation(xpos, lineheight * lineCount);
gv.setGlyphPosition(i, gmPos);
xpos = xpos + advance;
}
return gv;
}
}
View in GitHub: Java, KotlinExplanation
- 上:
JLabel
- デフォルトの
JLabel
で折り返しせずに右側から...
で省略
- デフォルトの
- 中:
GlyphVector
- コンテナのサイズが変更されるたびに
GlyphVector
を更新して文字列の折り返しを実行 - 欧文などで合字(リガチャ)がある場合は
GlyphVector gv = font.createGlyphVector(frc, str);
ではなく、GlyphVector bounds and kerning, ligatures | Oracle Forumsのようにchar[] chars = text.toCharArray(); GlyphVector gv = font.layoutGlyphVector(frc, chars, 0, chars.length, Font.LAYOUT_LEFT_TO_RIGHT);
とした方が良さそう
- コンテナのサイズが変更されるたびに
- 下:
JTextArea
JLabel
のFont
と背景色を同じものに設定した編集不可のJTextArea
をsetLineWrap(true);
として文字列の折り返しを実行
- ラベルの幅ではなく任意の場所で文字列を改行したい場合は、以下のように
JLabel
にhtml
の<br>
タグを利用したり、編集不可にしたJTextPane
、JTextArea
などが使用可能- 参考: JTextPane、JLabelなどで複数行を表示
label.setText("<html>文字列を適当なところで<br />折り返す。");
- 参考: JTextPane、JLabelなどで複数行を表示
AttributedString
とLineBreakMeasurer
を使って文字列の折り返しを描画する方法もあるclass WrappingLabel extends JLabel { public WrappingLabel(String text) { super(text); } @Override protected void paintComponent(Graphics g) { Graphics2D g2 = (Graphics2D) g.create(); g2.setPaint(getForeground()); Insets i = getInsets(); float x = i.left; float y = i.top; int w = getWidth() - i.left - i.right; AttributedString as = new AttributedString(getText()); as.addAttribute(TextAttribute.FONT, getFont()); AttributedCharacterIterator aci = as.getIterator(); FontRenderContext frc = g2.getFontRenderContext(); LineBreakMeasurer lbm = new LineBreakMeasurer(aci, frc); while (lbm.getPosition() < aci.getEndIndex()) { TextLayout tl = lbm.nextLayout(w); tl.draw(g2, x, y + tl.getAscent()); y += tl.getDescent() + tl.getLeading() + tl.getAscent(); } g2.dispose(); } }
Reference
- Drawing Multiple Lines of Text (The Java™ Tutorials > 2D Graphics > Working with Text APIs)
- LineBreakMeasurer (Java Platform SE 8)
- JDK-6479801 java.awt.font.LineBreakMeasurer code incorrect - Java Bug System