TransferHandlerを使ったJListのドラッグ&ドロップによる並べ替え
Total: 11141
, Today: 2
, Yesterday: 2
Posted by aterai at
Last-modified:
Summary
JList
のアイテムを複数選択し、ドラッグ&ドロップで並べ替えを可能にするTransferHandler
を作成します。
Screenshot
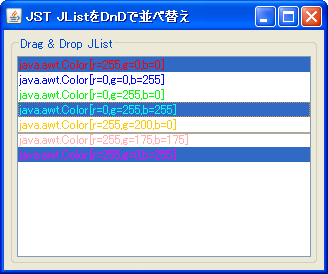
Advertisement
Source Code Examples
class ListItemTransferHandler extends TransferHandler {
protected final DataFlavor localObjectFlavor;
protected int[] indices;
protected int addIndex = -1; // Location where items were added
protected int addCount; // Number of items added.
protected ListItemTransferHandler() {
super();
localObjectFlavor = new DataFlavor(List.class, "List of items");
}
@Override protected Transferable createTransferable(JComponent c) {
JList<?> source = (JList<?>) c;
indices = source.getSelectedIndices();
List<?> transferredObjects = source.getSelectedValuesList();
return new Transferable() {
@Override public DataFlavor[] getTransferDataFlavors() {
return new DataFlavor[] {localObjectFlavor};
}
@Override public boolean isDataFlavorSupported(DataFlavor flavor) {
return Objects.equals(localObjectFlavor, flavor);
}
@Override public Object getTransferData(DataFlavor flavor)
throws UnsupportedFlavorException, IOException {
if (isDataFlavorSupported(flavor)) {
return transferredObjects;
} else {
throw new UnsupportedFlavorException(flavor);
}
}
};
}
@Override public boolean canImport(TransferSupport info) {
return info.isDrop() && info.isDataFlavorSupported(localObjectFlavor);
}
@Override public int getSourceActions(JComponent c) {
return TransferHandler.MOVE; // TransferHandler.COPY_OR_MOVE;
}
@SuppressWarnings("unchecked")
@Override public boolean importData(TransferHandler.TransferSupport info) {
TransferHandler.DropLocation tdl = info.getDropLocation();
if (!(tdl instanceof JList.DropLocation)) {
return false;
}
JList.DropLocation dl = (JList.DropLocation) tdl;
JList<?> target = (JList<?>) info.getComponent();
DefaultListModel listModel = (DefaultListModel) target.getModel();
// boolean insert = dl.isInsert();
int max = listModel.getSize();
int index = dl.getIndex();
// If it is out of range, it is appended to the end
index = index < 0 ? max : index;
index = Math.min(index, max);
addIndex = index;
try {
List<?> values = (List<?>) info.getTransferable()
.getTransferData(localObjectFlavor);
for (Object o : values) {
int i = index++;
listModel.add(i, o);
target.addSelectionInterval(i, i);
}
addCount = values.size();
return true;
} catch (UnsupportedFlavorException | IOException ex) {
ex.printStackTrace();
}
return false;
}
@Override protected void exportDone(
JComponent c, Transferable data, int action) {
cleanup(c, action == TransferHandler.MOVE);
}
private void cleanup(JComponent c, boolean remove) {
if (remove && Objects.nonNull(indices)) {
// If we are moving items around in the same list, we
// need to adjust the indices accordingly, since those
// after the insertion point have moved.
if (addCount > 0) {
for (int i = 0; i < indices.length; i++) {
if (indices[i] >= addIndex) {
indices[i] += addCount;
}
}
}
JList<?> source = (JList<?>) c;
DefaultListModel model = (DefaultListModel) source.getModel();
for (int i = indices.length - 1; i >= 0; i--) {
model.remove(indices[i]);
}
}
indices = null;
addCount = 0;
addIndex = -1;
}
}
View in GitHub: Java, KotlinExplanation
上記のサンプルのTransferHandler
は、主にDrag and Drop and Data Transfer: Examples (The Java™ Tutorials > Creating a GUI with JFC/Swing > Drag and Drop and Data Transfer)のListTransferHandler.javaを参考にして作成しています。ただし、このListTransferHandler.java
は項目を複数選択して、JList
内での並べ替えは想定していない(もしくはバグ?)ようなので、importData(...)
メソッドや、cleanup()
メソッドを修正しています。
JList list = new JList(listModel);
list.getSelectionModel().setSelectionMode(
ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
list.setTransferHandler(new ListItemTransferHandler());
list.setDropMode(DropMode.INSERT);
list.setDragEnabled(true);
importData
- 使用されていない?
importString(...)
の内容をこちらに移動
- 使用されていない?
cleanup
- 例えば、項目
0, 1, 2
を複数選択して1
と2
の間にドロップすると1, 2, 2
になる(0
が消えて2
になる)ので、以下のように修正for (int i = 0; i < indices.length; i++) { // if (indices[i] > addIndex) { if (indices[i] >= addIndex) { // ...
- 例えば、項目
JListの項目をドラッグ&ドロップとは異なり、複数アイテムを選択してDrag&Drop
による移動が可能になっています。
Reference
- Drag and Drop and Data Transfer: Examples (The Java™ Tutorials > Creating a GUI with JFC/Swing > Drag and Drop and Data Transfer)
- JListの項目をドラッグ&ドロップ
- JListのアイテムをラバーバンドで複数選択、ドラッグ&ドロップで並べ替え