Crossfadeで画像の切り替え
Total: 11287
, Today: 1
, Yesterday: 1
Posted by aterai at
Last-modified:
Summary
Crossfade
アニメーションで画像の切り替えを行います。
Screenshot
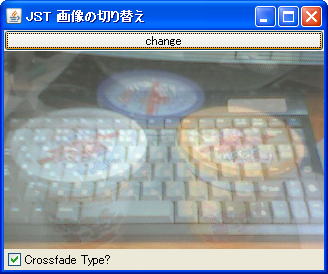
Advertisement
Source Code Examples
class Crossfade extends JComponent implements ActionListener {
private final javax.swing.Timer animator;
private final ImageIcon icon1;
private final ImageIcon icon2;
private int alpha = 10;
private boolean direction = true;
public Crossfade(ImageIcon icon1, ImageIcon icon2) {
this.icon1 = icon1;
this.icon2 = icon2;
animator = new javax.swing.Timer(50, this);
}
public void animationStart() {
direction = !direction;
animator.start();
}
@Override protected void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D) g.create();
g2.setPaint(getBackground());
g2.fillRect(0, 0, getWidth(), getHeight());
if (direction && alpha < 10) {
alpha = alpha + 1;
} else if (!direction && alpha > 0) {
alpha = alpha - 1;
} else {
animator.stop();
}
g2.setComposite(AlphaComposite.getInstance(
AlphaComposite.SRC_OVER, 1f - alpha * .1f));
g2.drawImage(icon1.getImage(), 0, 0,
(int) icon1.getIconWidth(), (int) icon1.getIconHeight(), this);
g2.setComposite(AlphaComposite.getInstance(
AlphaComposite.SRC_OVER, alpha * .1f));
g2.drawImage(icon2.getImage(), 0, 0,
(int) icon2.getIconWidth(), (int) icon2.getIconHeight(), this);
g2.dispose();
}
@Override public void actionPerformed(ActionEvent e) {
repaint();
}
}
View in GitHub: Java, KotlinExplanation
上記のサンプルでは、2
枚の画像の描画に使用するAlphaComposite
をそれぞれ変化させながら上書き(上書き規則にはAlphaComposite.SRC_OVERを使用)することで、画像の表示を切り替えています。