JTableのCellEditorに設定したJComboBoxに余白を追加する
Total: 6109
, Today: 1
, Yesterday: 2
Posted by aterai at
Last-modified:
概要
JTable
のCellEditor
に設定したJComboBox
に余白を追加します。
Screenshot
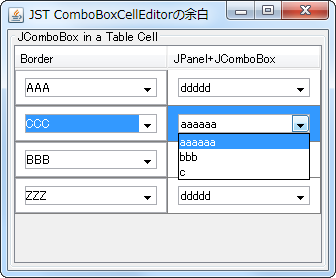
Advertisement
サンプルコード
class ComboBoxPanel extends JPanel {
public final JComboBox<String> comboBox = new JComboBox<>(
new String[] {"aaaaaa", "bbb", "c"});
public ComboBoxPanel() {
super(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.weightx = 1.0;
c.insets = new Insets(0, 10, 0, 10);
c.fill = GridBagConstraints.HORIZONTAL;
add(comboBox, c);
comboBox.setEditable(true);
comboBox.setSelectedIndex(0);
setOpaque(true);
}
}
class ComboBoxCellRenderer extends ComboBoxPanel implements TableCellRenderer {
public ComboBoxCellRenderer() {
super();
setName("Table.cellRenderer");
}
@Override public Component getTableCellRendererComponent(
JTable table, Object value,
boolean isSelected, boolean hasFocus, int row, int column) {
this.setBackground(isSelected ? table.getSelectionBackground()
: table.getBackground());
if (value != null) {
comboBox.setSelectedItem(value);
}
return this;
}
}
View in GitHub: Java, Kotlin解説
Border
(左)JComboBox
自身に余白を設定し、これをCellRenderer
とCellEditor
に適用- ドロップダウンリストの位置、サイズが余白を含んだ幅になる
combo.setBorder(BorderFactory.createCompoundBorder( BorderFactory.createEmptyBorder(8, 10, 8, 10), combo.getBorder()));
JPanel
+JComboBox
(右)GridBagLayout
を使用するJPanel
にJComboBox
を追加fill
フィールドをGridBagConstraints.HORIZONTAL
として垂直方向にはJComboBox
のサイズを変更しないinsets
フィールドを設定してJComboBox
の外側に別途(ドロップダウンリストの位置、サイズに影響しないように)余白を追加
セルの中にあるJComboBox
の幅を可変ではなく固定にする場合は、以下のようなFlowLayout
のパネルにgetPreferredSize()
をオーバーライドして幅を固定したJComboBox
を使用する方法もあります。
class ComboBoxPanel extends JPanel {
private String[] m = new String[] {"a", "b", "c"};
protected JComboBox<String> comboBox = new JComboBox<String>(m) {
@Override public Dimension getPreferredSize() {
Dimension d = super.getPreferredSize();
return new Dimension(40, d.height);
}
};
public ComboBoxPanel() {
super();
setOpaque(true);
comboBox.setEditable(true);
add(comboBox);
}
}