Swing/SortTree のバックアップ(No.27)
- バックアップ一覧
- 差分 を表示
- 現在との差分 を表示
- 現在との差分 - Visual を表示
- ソース を表示
- Swing/SortTree へ行く。
- 1 (2009-05-07 (木) 10:47:07)
- 2 (2010-03-08 (月) 12:21:42)
- 3 (2010-03-08 (月) 13:41:04)
- 4 (2012-10-28 (日) 22:23:20)
- 5 (2013-01-09 (水) 21:08:32)
- 6 (2013-07-02 (火) 15:53:41)
- 7 (2013-07-03 (水) 13:11:51)
- 8 (2013-07-03 (水) 18:16:27)
- 9 (2013-07-04 (木) 20:49:38)
- 10 (2013-07-10 (水) 13:59:51)
- 11 (2013-09-05 (木) 00:41:57)
- 12 (2013-09-05 (木) 17:56:17)
- 13 (2014-03-18 (火) 18:57:40)
- 14 (2014-10-07 (火) 20:28:39)
- 15 (2014-10-08 (水) 00:47:28)
- 16 (2014-11-13 (木) 01:39:43)
- 17 (2014-11-18 (火) 20:14:35)
- 18 (2014-11-18 (火) 21:21:24)
- 19 (2014-11-21 (金) 18:31:49)
- 20 (2014-11-22 (土) 03:48:33)
- 21 (2014-11-25 (火) 03:03:31)
- 22 (2015-02-18 (水) 15:11:40)
- 23 (2015-03-09 (月) 14:46:02)
- 24 (2015-03-16 (月) 17:28:33)
- 25 (2016-11-04 (金) 14:55:38)
- 26 (2017-03-29 (水) 13:55:51)
- 27 (2017-04-07 (金) 13:51:51)
- 28 (2018-02-13 (火) 16:00:29)
- 29 (2018-02-24 (土) 19:51:30)
- 30 (2019-05-22 (水) 19:35:38)
- 31 (2020-01-30 (木) 18:49:11)
- 32 (2021-07-29 (木) 02:58:44)
- 33 (2025-01-03 (金) 08:57:02)
- 34 (2025-01-03 (金) 09:01:23)
- 35 (2025-01-03 (金) 09:02:38)
- 36 (2025-01-03 (金) 09:03:21)
- 37 (2025-01-03 (金) 09:04:02)
- category: swing
folder: SortTree
title: JTreeのソート
tags: [JTree, TreeNode, Comparator]
author: aterai
pubdate: 2009-05-04T16:26:55+09:00
description: 葉ノードより親ノード優先でノード名を比較するComparatorを作成して、JTreeをソートします。
image:
hreflang:
href: http://java-swing-tips.blogspot.com/2013/09/how-to-sort-jtree-nodes.html lang: en
概要
葉ノードより親ノード優先でノード名を比較するComparator
を作成して、JTree
をソートします。
Screenshot
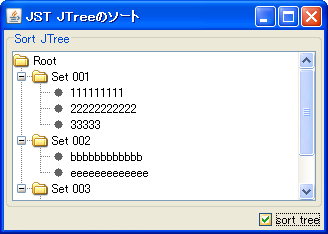
Advertisement
サンプルコード
public static void sortTree(DefaultMutableTreeNode root) {
Enumeration e = root.depthFirstEnumeration();
while (e.hasMoreElements()) {
DefaultMutableTreeNode node = (DefaultMutableTreeNode) e.nextElement();
if (!node.isLeaf()) {
sort2(node); //selection sort
//sort3(node); //JDK 1.6.0: iterative merge sort
//sort3(node); //JDK 1.7.0: TimSort
}
}
}
public static Comparator<DefaultMutableTreeNode> tnc = new Comparator<DefaultMutableTreeNode>() {
@Override public int compare(DefaultMutableTreeNode a, DefaultMutableTreeNode b) {
//...
}
};
View in GitHub: Java, Kotlin//selection sort
public static void sort2(DefaultMutableTreeNode parent) {
int n = parent.getChildCount();
for (int i = 0; i < n - 1; i++) {
int min = i;
for (int j = i + 1; j < n; j++) {
if (tnc.compare((DefaultMutableTreeNode) parent.getChildAt(min),
(DefaultMutableTreeNode) parent.getChildAt(j)) > 0) {
min = j;
}
}
if (i != min) {
MutableTreeNode a = (MutableTreeNode) parent.getChildAt(i);
MutableTreeNode b = (MutableTreeNode) parent.getChildAt(min);
parent.insert(b, i);
parent.insert(a, min);
}
}
}
public static void sort3(DefaultMutableTreeNode parent) {
int n = parent.getChildCount();
//@SuppressWarnings("unchecked")
//Enumeration<DefaultMutableTreeNode> e = parent.children();
//ArrayList<DefaultMutableTreeNode> children = Collections.list(e);
List<DefaultMutableTreeNode> children = new ArrayList<>(n);
for (int i = 0; i < n; i++) {
children.add((DefaultMutableTreeNode) parent.getChildAt(i));
}
Collections.sort(children, tnc); //using Arrays.sort(...)
parent.removeAllChildren();
for (MutableTreeNode node: children) {
parent.add(node);
}
}
解説
上記のサンプルでは、チェックボックスがクリックされると、以下の手順でソートを行っています。
DefaultTreeModel
からdeep copy
でクローンを作成- クローンされたモデルのルート
DefaultMutableTreeNode
を深さ優先で探索することで昇順ソート - ソート済みのモデルを
JTree
に設定- ソート無しの状態に戻す場合は、別途保存してある元の
DefaultTreeModel
をJTree
に設定
- ソート無しの状態に戻す場合は、別途保存してある元の
DefaultMutableTreeNode
の比較は、Comparator<DefaultMutableTreeNode>#compare
をオーバーライドし、節ノードが葉ノードより上、かつgetUserObject().toString()
で生成した文字列の大文字小文字を無視している
public static Comparator<DefaultMutableTreeNode> tnc = new Comparator<DefaultMutableTreeNode>() {
@Override public int compare(DefaultMutableTreeNode a, DefaultMutableTreeNode b) {
if (a.isLeaf() && !b.isLeaf()) {
return 1;
} else if (!a.isLeaf() && b.isLeaf()) {
return -1;
} else {
String sa = a.getUserObject().toString();
String sb = b.getUserObject().toString();
return sa.compareToIgnoreCase(sb);
}
}
};
JDK 1.8.0
以降の場合、このComparator
を以下のように簡単に作成できる
Comparator<String> sci = Comparator.comparingInt(String::length)
.thenComparing(String.CASE_INSENSITIVE_ORDER);
Comparator<DefaultMutableTreeNode> tnc = Comparator.comparing(DefaultMutableTreeNode::isLeaf)
.thenComparing(n -> n.getUserObject().toString(), sci);
// .thenComparing(n -> n.getUserObject().toString().toLowerCase());
sort3
で使用しているCollections.sort(...)
は、内部でArrays.sort(T[], Comparator<? super T>)
を使用しているので、JDK 1.6.0
と、JDK 1.7.0
以降でソートアルゴリズムが異なるようです(参考: Is Java 7 using Tim Sort for the Method Arrays.Sort? - Stack Overflow)。
JDK 1.6.0
//Arrays.sort(T[] a, Comparator<? super T> c) public static <T> void sort(T[] a, Comparator<? super T> c) { T[] aux = (T[]) a.clone(); if (c == null) { mergeSort(aux, a, 0, a.length, 0); } else { mergeSort(aux, a, 0, a.length, 0, c); } }
JDK 1.7.0
//Arrays.sort(T[] a, Comparator<? super T> c) public static <T> void sort(T[] a, Comparator<? super T> c) { if (c == null) { sort(a); } else { if (LegacyMergeSort.userRequested) { legacyMergeSort(a, c); } else { TimSort.sort(a, 0, a.length, c, null, 0, 0); } } }
参考リンク
- Swing - How to sort jTree Nodes
- 以下のコメントにバグの指摘あり
- JTreeのノードを走査する
JTree
ノードの深さ優先探索などについて
- JComboBoxのモデルとしてenumを使用する
- 各種ソートアルゴリズムのサンプル
- JTableでファイルとディレクトリを別々にソート
- ディレクトリが先になる比較について