JTreeのソート
Total: 9660
, Today: 1
, Yesterday: 1
Posted by aterai at
Last-modified:
Summary
JTree
を葉ノードより親ノード優先でノード名を比較するComparator
を使用してソートします。
Screenshot
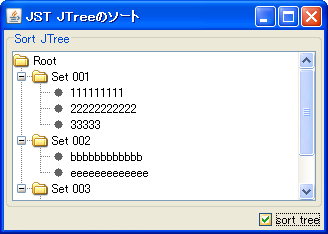
Advertisement
Source Code Examples
public static void sortTree(DefaultMutableTreeNode root) {
Enumeration e = root.depthFirstEnumeration();
while (e.hasMoreElements()) {
DefaultMutableTreeNode node = (DefaultMutableTreeNode) e.nextElement();
if (!node.isLeaf()) {
sort2(node); // selection sort
// sort3(node); // JDK 1.6.0: iterative merge sort
// sort3(node); // JDK 1.7.0: TimSort
}
}
}
public static Comparator<DefaultMutableTreeNode> tnc = new Comparator<DefaultMutableTreeNode>() {
@Override public int compare(DefaultMutableTreeNode a, DefaultMutableTreeNode b) {
// ...
}
};
View in GitHub: Java, Kotlin// selection sort
public static void sort2(DefaultMutableTreeNode parent) {
int n = parent.getChildCount();
for (int i = 0; i < n - 1; i++) {
int min = i;
for (int j = i + 1; j < n; j++) {
if (tnc.compare((DefaultMutableTreeNode) parent.getChildAt(min),
(DefaultMutableTreeNode) parent.getChildAt(j)) > 0) {
min = j;
}
}
if (i != min) {
MutableTreeNode a = (MutableTreeNode) parent.getChildAt(i);
MutableTreeNode b = (MutableTreeNode) parent.getChildAt(min);
parent.insert(b, i);
parent.insert(a, min);
}
}
}
public static void sort3(DefaultMutableTreeNode parent) {
int n = parent.getChildCount();
// @SuppressWarnings("unchecked")
// Enumeration<DefaultMutableTreeNode> e = parent.children();
// ArrayList<DefaultMutableTreeNode> children = Collections.list(e);
List<DefaultMutableTreeNode> children = new ArrayList<>(n);
for (int i = 0; i < n; i++) {
children.add((DefaultMutableTreeNode) parent.getChildAt(i));
}
Collections.sort(children, tnc); // using Arrays.sort(...)
parent.removeAllChildren();
for (MutableTreeNode node: children) {
parent.add(node);
}
}
Explanation
上記のサンプルでは、チェックボックスがクリックされると以下の手順でソートを実行します。
DefaultTreeModel
からdeep copy
でクローンを作成- クローンされたモデルのルート
DefaultMutableTreeNode
を深さ優先で探索することで昇順ソート - ソート済みのモデルを
JTree
に設定- ソート無しの状態に戻す場合は、別途保存してある元の
DefaultTreeModel
をJTree
に設定
- ソート無しの状態に戻す場合は、別途保存してある元の
DefaultMutableTreeNode
の比較はComparator<DefaultMutableTreeNode>#compare
をオーバーライドし、節ノードが葉ノードより上、かつgetUserObject().toString()
で生成した文字列の大文字小文字を無視している
public static Comparator<DefaultMutableTreeNode> tnc = new Comparator<DefaultMutableTreeNode>() {
@Override public int compare(DefaultMutableTreeNode a, DefaultMutableTreeNode b) {
if (a.isLeaf() && !b.isLeaf()) {
return 1;
} else if (!a.isLeaf() && b.isLeaf()) {
return -1;
} else {
String sa = a.getUserObject().toString();
String sb = b.getUserObject().toString();
return sa.compareToIgnoreCase(sb);
}
}
};
JDK 1.8.0
以降の場合、このComparator
を以下のように簡単に作成できる
Comparator<String> sci = Comparator.comparingInt(String::length)
.thenComparing(String.CASE_INSENSITIVE_ORDER);
Comparator<DefaultMutableTreeNode> tnc = Comparator.comparing(DefaultMutableTreeNode::isLeaf)
.thenComparing(n -> n.getUserObject().toString(), sci);
// .thenComparing(n -> n.getUserObject().toString().toLowerCase());
sort3
で使用しているCollections.sort(...)
は内部でArrays.sort(T[], Comparator<? super T>)
を使用しているので、JDK 1.6.0
とJDK 1.7.0
以降でソートアルゴリズムが異なる
JDK 1.6.0
// Arrays.sort(T[] a, Comparator<? super T> c) public static <T> void sort(T[] a, Comparator<? super T> c) { T[] aux = (T[]) a.clone(); if (c == null) { mergeSort(aux, a, 0, a.length, 0); } else { mergeSort(aux, a, 0, a.length, 0, c); } }
JDK 1.7.0
// Arrays.sort(T[] a, Comparator<? super T> c) public static <T> void sort(T[] a, Comparator<? super T> c) { if (c == null) { sort(a); } else { if (LegacyMergeSort.userRequested) { legacyMergeSort(a, c); } else { TimSort.sort(a, 0, a.length, c, null, 0, 0); } } }
Reference
- Swing - How to sort jTree Nodes
- 以下のコメントにバグの指摘あり
- JTreeのノードを走査する
JTree
ノードの深さ優先探索などについて
- JComboBoxのモデルとしてenumを使用する
- 各種ソートアルゴリズムのサンプル
- JTableでファイルとディレクトリを別々にソート
- ディレクトリが先になる比較について