TabComponentの名前を更新
Total: 6899
, Today: 1
, Yesterday: 3
Posted by aterai at
Last-modified:
Summary
TabComponent
を使用するJTabbedPane
で、タブ名称を編集更新します。
Screenshot
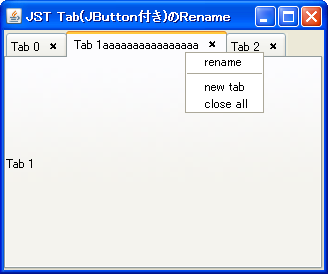
Advertisement
Source Code Examples
class TabTitleRenamePopupMenu extends JPopupMenu {
private final JTextField textField = new JTextField(10);
private final Action renameAction = new AbstractAction("rename") {
@Override public void actionPerformed(ActionEvent e) {
JTabbedPane t = (JTabbedPane) getInvoker();
int idx = t.getSelectedIndex();
String title = t.getTitleAt(idx);
textField.setText(title);
int result = JOptionPane.showConfirmDialog(t, textField, "Rename",
JOptionPane.OK_CANCEL_OPTION, JOptionPane.PLAIN_MESSAGE);
if (result == JOptionPane.OK_OPTION) {
String str = textField.getText();
if (!str.trim().isEmpty()) {
t.setTitleAt(idx, str);
JComponent c = (JComponent) t.getTabComponentAt(idx);
c.revalidate();
}
}
}
};
private final Action newTabAction = new AbstractAction("new tab") {
@Override public void actionPerformed(ActionEvent evt) {
JTabbedPane t = (JTabbedPane) getInvoker();
int count = t.getTabCount();
String title = "Tab " + count;
t.add(title, new JLabel(title));
t.setTabComponentAt(count, new ButtonTabComponent(t));
}
};
private final Action closeAllAction = new AbstractAction("close all") {
@Override public void actionPerformed(ActionEvent evt) {
JTabbedPane t = (JTabbedPane) getInvoker();
t.removeAll();
}
};
public TabTitleRenamePopupMenu() {
super();
textField.addAncestorListener(new AncestorListener() {
@Override public void ancestorAdded(AncestorEvent e) {
textField.requestFocusInWindow();
}
@Override public void ancestorMoved(AncestorEvent e) {}
@Override public void ancestorRemoved(AncestorEvent e) {}
});
add(renameAction);
addSeparator();
add(newTabAction);
add(closeAllAction);
}
@Override public void show(Component c, int x, int y) {
JTabbedPane t = (JTabbedPane) c;
renameAction.setEnabled(t.indexAtLocation(x, y) >= 0);
super.show(c, x, y);
}
};
View in GitHub: Java, KotlinExplanation
- タブを閉じる
JButton
をTabComponent
に追加したJTabbedPane
にタブ名称を変更するJPopupMenu
を設定 - How to Use Tabbed Panes (The Java™ Tutorials > Creating a GUI With JFC/Swing > Using Swing Components)の
ButtonTabComponent
を使用JTabbedPane#setTitleAt(...)
メソッドで名前を変更したときにtabbedPane.getTabComponentAt(idx)
で取得したJComponent
をrevalidate()
することで文字列の長さに応じたサイズへの変更とタブの内部レイアウトの更新を実行
Reference
- How to Use Tabbed Panes (The Java™ Tutorials > Creating a GUI With JFC/Swing > Using Swing Components)
- JTabbedPaneのタブにJTextFieldを配置してタイトルを編集
- JTabbedPaneのタブタイトルを変更
- JTabbedPaneにタブを閉じるボタンを追加