HTMLEditorKitを適用したJEditorPaneのフォームにコンポーネントを表示する
Total: 1682
, Today: 3
, Yesterday: 3
Posted by aterai at
Last-modified:
Summary
HTMLEditorKit
を適用したJEditorPane
のフォームにinput
やselect
タグで表示可能なコンポーネントをテストします。
Screenshot
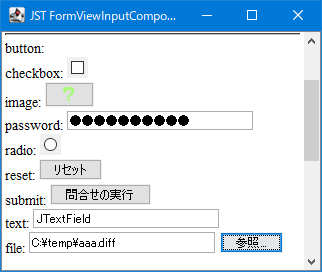
Advertisement
Source Code Examples
JEditorPane editor = new JEditorPane();
HTMLEditorKit kit = new HTMLEditorKit();
kit.setAutoFormSubmission(false);
editor.setEditorKit(kit);
editor.setEditable(false);
editor.addHyperlinkListener(e -> {
if (e instanceof FormSubmitEvent) {
String data = ((FormSubmitEvent) e).getData();
String charset = Charset.defaultCharset().toString();
try {
System.out.println(URLDecoder.decode(data, charset));
} catch (UnsupportedEncodingException ex) {
UIManager.getLookAndFeel().provideErrorFeedback(editor);
ex.printStackTrace();
}
}
});
editor.setText(makeHtml());
// ...
private static String makeHtml() {
ClassLoader cl = Thread.currentThread().getContextClassLoader();
String path = "example/16x16.png";
String src = Optional.ofNullable(cl.getResource(path)).map(URL::toString).orElse("not found");
// https://docs.oracle.com/javase/8/docs/api/javax/swing/text/html/FormView.html
return String.join(
"\n",
"<html><body><form id='form1' action='#'>",
"<div>Username: <input type='text' id='username' name='username'></div>",
"<div>Password: <input type='password' id='password' name='password'></div>",
"<input type='submit' value='Submit'>",
"</form><br/><hr/>",
"<form id='form2' action='#'>",
"<div>button: <input type='button' value='JButton'></div>",
"<div>checkbox: <input type='checkbox' id='checkbox1' name='checkbox1'></div>",
"<div>image: <input type='image' id='image1' name='image1' src='" + src + "'></div>",
"<div>password: <input type='password' id='password1' name='password1'></div>",
"<div>radio: <input type='radio' id='radio1' name='radio1'></div>",
"<div>reset: <input type='reset' id='reset1' name='reset1'></div>",
"<div>submit: <input type='submit' id='submit1' name='submit1'></div>",
"<div>text: <input type='text' id='text1' name='text1'></div>",
"<div>file: <input type='file' id='file1' name='file1'></div>",
"<div><isindex id='search1' name='search1' action='#'></div>",
"<div><isindex id='search2' name='search2' prompt='search: ' action='#'></div>",
"</form><br/><hr/>",
"<form id='form3' action='#'>",
"<div><select name='select1' size='5' multiple>",
" <option value='' selected='selected'>selected</option>",
" <option value='option1'>option1</option>",
" <option value='option2'>option2</option>",
"</select></div><br/>",
"<div><select name='select2'>",
" <option value='option0'>option0</option>",
" <option value='option1'>option1</option>",
" <option value='option2'>option2</option>",
"</select></div><br/>",
"<div><textarea name='textarea1' cols='50' rows='5'></div>",
"</form>",
"</body></html>");
}
View in GitHub: Java, KotlinExplanation
<input type='button' ...>
空白になるJButton
が使用されるtype
属性がbutton
の場合はドキュメントに記述されているがソースコードでは実装されていない
<input type='checkbox' ...>
JCheckBox
が使用されるchecked
属性で選択状態になる
<input type='radio' ...>
JRadioButton
が使用されるchecked
属性で選択状態になる
<input type='image' ...>
JButton
が使用されるsrc
属性で画像ファイルのURL
が指定可能- クリック座標が取得可能
<input type='password' ...>
JPasswordField
が使用される
<input type='text' ...>
JTextField
が使用される
<input type='reset' ...>
JButton
が使用されるvalue
属性でボタンラベルが変更可能
<input type='submit' ...>
JButton
が使用されるvalue
属性でボタンラベルが変更可能
<input type='file' ...>
JTextField
とJButton
が使用されるJButton
をクリックするとJFileChooser
が開き、選択したファイルのフルパスがJTextField
に表示される
<isindex prompt='...' action='#' ...>
JLabel
とJTextField
が使用されるJLabel
のテキストはprompt
属性で指定、またはUIManager.put("IsindexView.prompt", "Search: ")
などで設定可能
<select ...>
size
属性が1
以上、またはmultiple
属性が存在する場合はJScrollPane
内に配置されたJList
が使用される
<select ...>
size
属性が1
、または存在しない場合はJComboBox
が使用される
<textarea ...>
JScrollPane
内に配置されたJTextArea
が使用されるrows
、cols
属性でJTextArea
の行数と列数を指定可能
Reference
- FormView (Java Platform SE 8 )
- 日本語ドキュメントでは
select
要素、size
属性、multiple
属性などが次のように翻訳されてしまい少しわかりづらい - 「選択、サイズは> 1、または複数の属性を定義」
- 日本語ドキュメントでは
- JEditorPaneに表示されたフォームからの送信データを取得する