JTableのセルのハイライト
Total: 17583
, Today: 1
, Yesterday: 6
Posted by aterai at
Last-modified:
Summary
JTable
のセル上にマウスカーソルが存在する場合、その背景色を変更します。
Screenshot
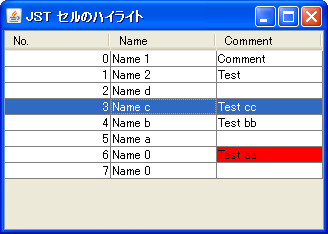
Advertisement
Source Code Examples
class HighlightListener extends MouseAdapter {
private int row = -1;
private int col = -1;
private final JTable table;
public HighlightListener(JTable table) {
this.table = table;
}
public boolean isHighlightableCell(int row, int column) {
return this.row == row && this.col == column;
}
@Override public void mouseMoved(MouseEvent e) {
Point pt = e.getPoint();
row = table.rowAtPoint(pt);
col = table.columnAtPoint(pt);
if (row < 0 || col < 0) {
row = -1;
col = -1;
}
table.repaint();
}
@Override public void mouseExited(MouseEvent e) {
row = -1;
col = -1;
table.repaint();
}
}
class HighlightRenderer extends DefaultTableCellRenderer {
private final HighlightListener highlighter;
public HighlightRenderer(JTable table) {
super();
highlighter = new HighlightListener(table);
table.addMouseListener(highlighter);
table.addMouseMotionListener(highlighter);
}
@Override public Component getTableCellRendererComponent(JTable table,
Object value, boolean isSelected, boolean hasFocus,
int row, int column) {
super.getTableCellRendererComponent(
table, value, isSelected, hasFocus, row, column);
setHorizontalAlignment((value instanceof Number) ? RIGHT : LEFT);
if (highlighter.isHighlightableCell(row, column)) {
setBackground(Color.RED);
} else {
setBackground(isSelected ? table.getSelectionBackground()
: table.getBackground());
}
return this;
}
}
View in GitHub: Java, KotlinExplanation
上記のサンプルでは、JTable
にMouseListener
、MouseMotionListener
を追加してマウスカーソルが乗っているセルを取得し、セルレンダラーでこれを参照してその背景色を変更しています。
JTable#prepareRenderer(...)
メソッドをオーバーライドする方法もあるclass HighlightableTable extends JTable { private final HighlightListener highlighter; public HighlightableTable(TableModel model) { super(model); highlighter = new HighlightListener(this); addMouseListener(highlighter); addMouseMotionListener(highlighter); } @Override public Component prepareRenderer( TableCellRenderer r, int row, int column) { Component c = super.prepareRenderer(r, row, column); if (highlighter.isHighlightableCell(row, column)) { c.setBackground(Color.RED); } else if (isRowSelected(row)) { c.setBackground(getSelectionBackground()); } else { c.setBackground(Color.WHITE); } return c; } }