Swing/NineSliceScalingButton のバックアップ(No.4)
- バックアップ一覧
- 差分 を表示
- 現在との差分 を表示
- 現在との差分 - Visual を表示
- ソース を表示
- Swing/NineSliceScalingButton へ行く。
- 1 (2013-08-12 (月) 00:58:18)
- 2 (2013-08-12 (月) 15:12:25)
- 3 (2014-11-22 (土) 03:59:17)
- 4 (2014-11-22 (土) 16:15:37)
- 5 (2016-01-05 (火) 15:56:47)
- 6 (2017-06-16 (金) 14:13:28)
- 7 (2017-11-29 (水) 15:40:06)
- 8 (2018-02-24 (土) 19:51:30)
- 9 (2019-07-18 (木) 16:50:40)
- 10 (2019-08-15 (木) 14:43:47)
- 11 (2021-04-14 (水) 04:02:37)
- title: JButtonに9分割した画像を使用する
tags: [JButton, Icon, BufferedImage, RGBImageFilter]
author: aterai
pubdate: 2013-08-12T00:58:18+09:00
description: JButtonを拡大縮小しても四隅などのサイズが変更しないようにように9分割した画像を使用します。
hreflang:
href: http://java-swing-tips.blogspot.com/2013/08/create-9-slice-scaling-image-jbutton.html lang: en
概要
JButton
を拡大縮小しても四隅などのサイズが変更しないようにように9
分割した画像を使用します。
Screenshot
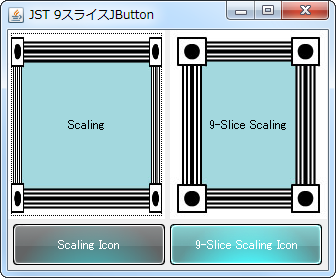
Advertisement
サンプルコード
class NineSliceScalingIcon implements Icon {
private final BufferedImage image;
private final int a, b, c, d;
private int width, height;
public NineSliceScalingIcon(BufferedImage image, int a, int b, int c, int d) {
this.image = image;
this.a = a;
this.b = b;
this.c = c;
this.d = d;
}
@Override public int getIconWidth() {
return width;
}
@Override public int getIconHeight() {
return Math.max(image.getHeight(null), height);
}
@Override public void paintIcon(Component cmp, Graphics g, int x, int y) {
Graphics2D g2 = (Graphics2D) g.create();
g2.setRenderingHint(
RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
Insets i = ((JComponent) cmp).getBorder().getBorderInsets(cmp);
//g2.translate(x, y); //JDK 1.7.0 などで描画がおかしい?
int iw = image.getWidth(cmp);
int ih = image.getHeight(cmp);
width = cmp.getWidth()-i.left-i.right;
height = cmp.getHeight()-i.top-i.bottom;
g2.drawImage(image.getSubimage(a, c, iw - a - b, ih - c - d),
a, c, width - a - b, height - c - d, cmp);
if(a>0 && b>0 && c>0 && d>0) {
g2.drawImage(image.getSubimage(a, 0, iw - a - b, c),
a, 0, width - a - b, c, cmp);
g2.drawImage(image.getSubimage(a, ih - d, iw - a - b, d),
a, height - d, width - a - b, d, cmp);
g2.drawImage(image.getSubimage(0, c, a, ih - c - d),
0, c, a, height - c - d, cmp);
g2.drawImage(image.getSubimage(iw - b, c, b, ih - c - d),
width - b, c, b, height - c - d, cmp);
g2.drawImage(image.getSubimage(0, 0, a, c), 0, 0, cmp);
g2.drawImage(image.getSubimage(iw - b, 0, b, c), width - b, 0, cmp);
g2.drawImage(image.getSubimage(0, ih - d, a, d), 0, height - d, cmp);
g2.drawImage(image.getSubimage(iw - b, ih - d, b, d), width - b, height - d, cmp);
}
g2.dispose();
}
}
View in GitHub: Java, Kotlin解説
上記のサンプルでは、BufferedImage#getSubimage
で元画像を9
分割し、四隅はサイズ変更なし、上下辺は幅のみ拡大縮小、左右辺は高さのみ拡大縮小、中央は幅高さが拡大縮小可能になるよう、Graphics#drawImage(...)
のスケーリングを利用して描画しています。
- 四隅などの固定サイズ
a
: 左上下隅の幅b
: 右上下隅の幅c
: 左右上隅の高さd
: 左右下隅の高さ
参考リンク
- Real World Illustrator: Understanding 9-Slice Scaling
- テスト用の画像(
symbol_scale_2.jpg
)を拝借しています。
- テスト用の画像(