JTextPaneのStyledDocumentからhtmlテキストを生成する
Total: 825
, Today: 3
, Yesterday: 1
Posted by aterai at
Last-modified:
Summary
JTextPane
から取得したStyledDocument
をMinimalHTMLWriter
で変換してhtml
テキストを生成します。
Screenshot
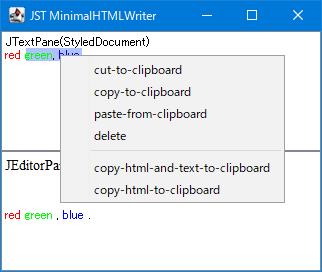
Advertisement
Source Code Examples
public void copyHtmlTextToClipboard(JTextPane textPane) {
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
int start = textPane.getSelectionStart();
int end = textPane.getSelectionEnd();
int length = end - start;
StyledDocument styledDocument = textPane.getStyledDocument();
try (OutputStream os = new ByteArrayOutputStream();
OutputStreamWriter writer = new OutputStreamWriter(
os, StandardCharsets.UTF_8)) {
MinimalHTMLWriter w = new MinimalHTMLWriter(
writer, styledDocument, start, length);
w.write();
writer.flush();
String contents = os.toString();
String plain= styledDocument .getText(start, length);
Transferable transferable = new BasicTransferable(plain, contents);
clipboard.setContents(transferable, null);
} catch (IOException | BadLocationException e) {
UIManager.getLookAndFeel().provideErrorFeedback(textPane);
e.printStackTrace();
}
}
View in GitHub: Java, KotlinExplanation
copy-to-clipboard
DefaultEditorKit.CopyAction()
を使用して選択テキストをコピー- Ctrl+Cでのコピーと同じ
- コピー元の
JTextPane
にペーストすればDataFlavor
がapplication/x-java-jvm-local-objectref
に対応しているのでスタイルを維持したテキストになる JEditorPane#setContentType("text/html")
を設定してHTMLEditorKit
を使用するJEditorPane
にペーストするとスタイルは除去されたテキストになる
copy-html-and-text-to-clipboard
MinimalHTMLWriter
を使用してスタイル付きテキストをHtml
テキストに変換してクリップボードにコピーjavax/swing/plaf/basic/BasicTransferable.java
をコピーしてプレーンテキストとHtml
テキストを保持するTransferable
を生成してクリップボードにコピー- このため
JEditorPane#setContentType("text/html")
を設定してHTMLEditorKit
を使用するJEditorPane
にHtml
テキストとしてペーストが可能 MinimalHTMLWriter
でHtml
化するとタグ間に空白文字が追加されたり、改行文字が追加・除去される場合がある?
HTMLEditorKit#write(Writer out, Document doc, int pos, int len)
では、以下のようにHTMLDocument
はHTMLWriter
で、StyledDocument
はMinimalHTMLWriter
でhtml
テキストに変換しているのでこれを参考にしている
public void write(Writer out, Document doc, int pos, int len)
throws IOException, BadLocationException {
if (doc instanceof HTMLDocument) {
HTMLWriter w = new HTMLWriter(out, (HTMLDocument) doc, pos, len);
w.write();
} else if (doc instanceof StyledDocument) {
MinimalHTMLWriter w = new MinimalHTMLWriter(out, (StyledDocument) doc, pos, len);
w.write();
} else {
super.write(out, doc, pos, len);
}
}
copy-html-to-clipboard
copy-html-and-text-to-clipboard
と同様にMinimalHTMLWriter
を使用してスタイル付きテキストをHtml
テキストに変換し、以下のようなHtml
テキストのみを保持する簡易Transferable
を生成してクリップボードにコピーJEditorPane#setContentType("text/html")
を設定してHTMLEditorKit
を使用するJEditorPane
にHtml
テキストとしてペーストが可能だが、コピー元のJTextPane
にペースト不可になる
class HtmlTransferable implements Transferable {
private final String htmlFormattedText;
public HtmlTransferable(String htmlFormattedText) {
this.htmlFormattedText = htmlFormattedText;
}
@Override public DataFlavor[] getTransferDataFlavors() {
return new DataFlavor[] { DataFlavor.allHtmlFlavor };
}
@Override public boolean isDataFlavorSupported(DataFlavor flavor) {
for (DataFlavor supportedFlavor : getTransferDataFlavors()) {
if (supportedFlavor.equals(flavor)) {
return true;
}
}
return false;
}
@Override public Object getTransferData(DataFlavor flavor)
throws UnsupportedFlavorException, IOException {
if (Objects.equals(flavor, DataFlavor.allHtmlFlavor)) {
return htmlFormattedText;
}
throw new UnsupportedFlavorException(flavor);
}
}
Reference
- MinimalHTMLWriter (Java Platform SE 8)
- JTextPaneで修飾したテキストをJTextAreaにHtmlソースとして表示する
- JTextPaneでキーワードのSyntaxHighlight