Swing/ScrollIncrement のバックアップ(No.19)
- バックアップ一覧
- 差分 を表示
- 現在との差分 を表示
- 現在との差分 - Visual を表示
- ソース を表示
- Swing/ScrollIncrement へ行く。
- 1 (2004-03-11 (木) 15:48:27)
- 2 (2004-06-02 (水) 09:59:28)
- 3 (2004-07-23 (金) 10:38:07)
- 4 (2004-09-08 (水) 09:54:06)
- 5 (2004-09-22 (水) 05:54:38)
- 6 (2004-10-08 (金) 06:24:47)
- 7 (2004-11-04 (木) 10:11:00)
- 8 (2005-04-28 (木) 04:32:57)
- 9 (2005-10-06 (木) 10:43:07)
- 10 (2006-02-27 (月) 16:21:39)
- 11 (2007-03-11 (日) 22:32:23)
- 12 (2007-10-06 (土) 21:31:14)
- 13 (2012-07-10 (火) 20:57:35)
- 14 (2013-02-26 (火) 14:47:10)
- 15 (2013-02-26 (火) 15:55:03)
- 16 (2013-05-03 (金) 23:46:03)
- 17 (2013-05-26 (日) 05:58:34)
- 18 (2013-08-17 (土) 00:23:15)
- 19 (2014-12-28 (日) 15:25:28)
- 20 (2015-02-18 (水) 15:07:25)
- 21 (2015-02-26 (木) 14:03:46)
- 22 (2015-03-19 (木) 16:29:29)
- 23 (2015-06-26 (金) 17:48:10)
- 24 (2016-11-01 (火) 19:45:31)
- 25 (2017-04-04 (火) 14:17:08)
- 26 (2017-11-02 (木) 15:32:16)
- 27 (2017-11-17 (金) 18:11:07)
- 28 (2019-07-08 (月) 18:10:07)
- 29 (2021-03-19 (金) 00:44:39)
- 30 (2025-01-03 (金) 08:57:02)
- 31 (2025-01-03 (金) 09:01:23)
- 32 (2025-01-03 (金) 09:02:38)
- 33 (2025-01-03 (金) 09:03:21)
- 34 (2025-01-03 (金) 09:04:02)
- 35 (2025-06-19 (木) 12:41:37)
- 36 (2025-06-19 (木) 12:43:47)
- title: JScrollPaneのスクロール量を変更 tags: [JScrollPane, JScrollBar] author: aterai pubdate: 2003-12-01 description: スクロールがホイールの回転でスムーズに移動しない(遅い)場合は、JScrollPaneのスクロール量を変更します。
概要
スクロールがホイールの回転でスムーズに移動しない(遅い)場合は、JScrollPane
のスクロール量を変更します。
Screenshot
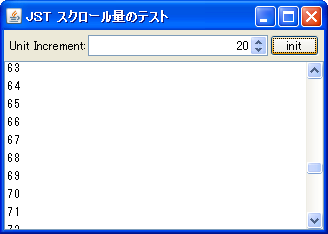
Advertisement
サンプルコード
JScrollPane scroll = new JScrollPane();
scroll.getVerticalScrollBar().setUnitIncrement(25);
View in GitHub: Java, Kotlin解説
JScrollPane
からスクロールバーを取得し、JScrollBar#setUnitIncrement(int)
メソッドでユニット増分値(unitIncrement
)を設定しています。
メモ: JScrollBar
のUnitIncrement
とBlockIncrement
について
- Mouse Wheel Controller « Java Tips Weblog
Windows 7
環境でのe.getScrollAmount()
のデフォルト値:3
DesktopProperty
などを経由せず、OS
のコントロールパネルのマウスのプロパティを直接参照している模様?
- How to Write a Mouse-Wheel Listener (The Java™ Tutorials > Creating a GUI With JFC/Swing > Writing Event Listeners)
scrollPane.getVerticalScrollBar().setBlockIncrement(...)
を設定した場合、その設定した値とScroll amount
(デフォルトの3
固定) ×unit increment(scrollPane.getVerticalScrollBar().getUnitIncrement(...))
のより小さい方がホイールでのスクロール量となる- ただし、
scrollPane.getVerticalScrollBar().setBlockIncrement(...)
で設定した値が、scrollPane.getVerticalScrollBar().getUnitIncrement(...)
より小さい場合は、UnitIncrement
自体が、ホイールでのスクロール量となる - このため、例えば縦のアイテムを配置する通常の
JList
などのホイールスクロールをスクロールバーの矢印ボタンクリックと同じ1
行ごとにしたいなら、scrollPane.getVerticalScrollBar().setBlockIncrement(0)
でも可能 - すでに上限、下限近くまでスクロールして余地がない場合などは除く(限界を超えてスクロールなどはしない)
- ただし、
Scrollable#getScrollableUnitIncrement(...)
などをオーバーライドしても、ホイールスクロールには関係ない?(カーソルキー用?)JScrollBar#getClientProperty("JScrollBar.fastWheelScrolling")
がtrue
(デフォルト)で、View
がJList
などのようにScrollable
を実装している場合は、Scrollable#getScrollableUnitIncrement(...)
、Scrollable#getScrollableBlockIncrement(...)
が使用されている@See BasicScrollPaneUI.Handler
- マウスのプロパティで「
1
画面ずつスクロールする」にすると、e.getScrollType()
がWHEEL_UNIT_SCROLL
からWHEEL_BLOCK_SCROLL
になり、上記の3
行×スクロール量(BasicScrollBarUI.scrollByUnits(...)
)ではなく、BasicScrollBarUI.scrollByBlock(...)
が使用される
//package example;
//-*- mode:java; encoding:utf8n; coding:utf-8 -*-
// vim:set fileencoding=utf-8:
//@homepage@
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
public class Bbb extends JPanel{
public Bbb() {
super(new BorderLayout());
JList list = makeList();
final JScrollPane scrollPane = new JScrollPane(list) {
@Override protected void processMouseWheelEvent(MouseWheelEvent e) {
MouseWheelEvent mwe = new MouseWheelEvent(
e.getComponent(),
e.getID(),
e.getWhen(),
e.getModifiers(),
e.getX(), e.getY(),
e.getXOnScreen(),
e.getYOnScreen(),
e.getClickCount(), //???Nimbusを追加???
e.isPopupTrigger(),
e.getScrollType(),
100, //e.getScrollAmount(),
e.getWheelRotation());
super.processMouseWheelEvent(mwe);
}
};
scrollPane.addMouseWheelListener(new MouseWheelListener() {
@Override public void mouseWheelMoved(MouseWheelEvent e) {
//System.out.println(e);
if (e.getScrollType() == MouseWheelEvent.WHEEL_UNIT_SCROLL) {
System.out.println("WHEEL_UNIT_SCROLL");
}else if (e.getScrollType() == MouseWheelEvent.WHEEL_BLOCK_SCROLL) {
System.out.println("WHEEL_BLOCK_SCROLL");
}
}
});
list.setFixedCellHeight(64);
//System.out.println(list.getFixedCellHeight());
scrollPane.getVerticalScrollBar().setUnitIncrement(32);
scrollPane.getVerticalScrollBar().setBlockIncrement(64*6);
JViewport vp = scrollPane.getViewport();
Scrollable view = (Scrollable)(vp.getView());
Rectangle vr = vp.getViewRect();
System.out.println("getUnitIncrement: "+scrollPane.getVerticalScrollBar().getUnitIncrement(1));
System.out.println("getBlockIncrement: "+scrollPane.getVerticalScrollBar().getBlockIncrement(1));
System.out.println("getScrollableUnitIncrement: "+view.getScrollableUnitIncrement(vr, scrollPane.getVerticalScrollBar().getOrientation(), 1));
System.out.println("getScrollableBlockIncrement: "+view.getScrollableBlockIncrement(vr, scrollPane.getVerticalScrollBar().getOrientation(), 1));
scrollPane.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
final JSpinner spinner = new JSpinner(new SpinnerNumberModel(scrollPane.getVerticalScrollBar().getBlockIncrement(1), 0, 100000, 1));
spinner.setEditor(new JSpinner.NumberEditor(spinner, "#####0"));
spinner.addChangeListener(new ChangeListener() {
@Override public void stateChanged(ChangeEvent e) {
JSpinner s = (JSpinner)e.getSource();
Integer iv = (Integer)s.getValue();
System.out.println(iv);
scrollPane.getVerticalScrollBar().setBlockIncrement(iv);
}
});
Box box = Box.createHorizontalBox();
box.add(new JLabel("Block Increment:"));
box.add(Box.createHorizontalStrut(2));
box.add(spinner);
box.setBorder(BorderFactory.createEmptyBorder(5,5,5,5));
add(box, BorderLayout.NORTH);
add(scrollPane);
setPreferredSize(new Dimension(320, 200));
}
@SuppressWarnings("unchecked")
private static JList makeList() {
DefaultListModel model = new DefaultListModel();
for(int i=0;i<100;i++) {
model.addElement("Item: "+i);
}
JList list = new JList(model) {
// @Override public int getScrollableUnitIncrement(Rectangle visibleRect, int orientation, int direction) {
// return 64;
// }
// @Override public int getScrollableBlockIncrement(Rectangle visibleRect, int orientation, int direction) {
// if (orientation == SwingConstants.VERTICAL) {
// int inc = visibleRect.height;
// /* Scroll Down */
// if (direction > 0) {
// // last cell is the lowest left cell
// int last = locationToIndex(new Point(visibleRect.x, visibleRect.y+visibleRect.height-1));
// if (last != -1) {
// Rectangle lastRect = getCellBounds(last,last);
// if (lastRect != null) {
// inc = lastRect.y - visibleRect.y;
// if ( (inc == 0) && (last < getModel().getSize()-1) ) {
// inc = lastRect.height;
// }
// }
// }
// }
// /* Scroll Up */
// else {
// int newFirst = locationToIndex(new Point(visibleRect.x, visibleRect.y-visibleRect.height));
// int first = getFirstVisibleIndex();
// if (newFirst != -1) {
// if (first == -1) {
// first = locationToIndex(visibleRect.getLocation());
// }
// Rectangle newFirstRect = getCellBounds(newFirst,newFirst);
// Rectangle firstRect = getCellBounds(first,first);
// if ((newFirstRect != null) && (firstRect!=null)) {
// while ( (newFirstRect.y + visibleRect.height <
// firstRect.y + firstRect.height) &&
// (newFirstRect.y < firstRect.y) ) {
// newFirst++;
// newFirstRect = getCellBounds(newFirst,newFirst);
// }
// inc = visibleRect.y - newFirstRect.y;
// if ( (inc <= 0) && (newFirstRect.y > 0)) {
// newFirst--;
// newFirstRect = getCellBounds(newFirst,newFirst);
// if (newFirstRect != null) {
// inc = visibleRect.y - newFirstRect.y;
// }
// }
// }
// }
// }
// return 128;
// }
// return super.getScrollableBlockIncrement(visibleRect, orientation, direction);
// }
};
list.setCellRenderer(new DefaultListCellRenderer() {
private final Color ec = new Color(240, 240, 240);
@Override public Component getListCellRendererComponent(JList list, Object value, int index, boolean isSelected, boolean cellHasFocus) {
super.getListCellRendererComponent(list,value,index,isSelected,cellHasFocus);
if(isSelected) {
setForeground(list.getSelectionForeground());
setBackground(list.getSelectionBackground());
}else{
setForeground(list.getForeground());
setBackground(((index%2==0))?ec:list.getBackground());
}
return this;
}
});
return list;
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override public void run() {
createAndShowGUI();
}
});
}
public static void createAndShowGUI() {
// try{
// UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
// }catch(Exception e) {
// e.printStackTrace();
// }
JFrame frame = new JFrame("@title@");
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.getContentPane().add(new Bbb());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}