JFrameの位置・サイズを記憶する
Total: 25428
, Today: 1
, Yesterday: 3
Posted by aterai at
Last-modified:
Summary
Preferences
(レジストリなど)にフレーム(パネル)の位置・サイズを記憶しておきます。
Screenshot
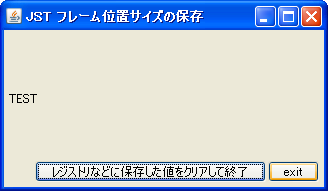
Advertisement
Source Code Examples
public MainPanel(JFrame frame) {
super(new BorderLayout());
this.setBorder(BorderFactory.createEmptyBorder(5, 5, 5, 5));
this.prefs = Preferences.userNodeForPackage(getClass());
frame.addWindowListener(new WindowAdapter() {
@Override public void windowClosing(WindowEvent e) {
saveLocation();
e.getWindow().dispose();
}
});
frame.addComponentListener(new ComponentAdapter() {
@Override public void componentMoved(ComponentEvent e) {
JFrame frame = (JFrame) e.getComponent();
if (frame.getExtendedState() == Frame.NORMAL) {
Point pt = frame.getLocationOnScreen();
if (pt.x < 0 || pt.y < 0) {
return;
}
try {
pos.setLocation(pt);
} catch (IllegalComponentStateException icse) {
icse.printStackTrace();
}
}
}
@Override public void componentResized(ComponentEvent e) {
JFrame frame = (JFrame) e.getComponent();
if (frame.getExtendedState() == Frame.NORMAL) {
dim.setSize(getSize());
}
}
});
Box box = Box.createHorizontalBox();
box.add(Box.createHorizontalGlue());
box.add(clearButton);
box.add(Box.createHorizontalStrut(2));
box.add(exitButton);
add(new JLabel("TEST"));
add(box, BorderLayout.SOUTH);
int wdim = prefs.getInt(PREFIX + "dimw", dim.width);
int hdim = prefs.getInt(PREFIX + "dimh", dim.height);
dim.setSize(wdim, hdim);
setPreferredSize(dim);
Rectangle screen = frame.getGraphicsConfiguration().getBounds();
pos.setLocation(screen.x + screen.width / 2 - dim.width / 2,
screen.y + screen.height / 2 - dim.height / 2);
int xpos = prefs.getInt(PREFIX + "locx", pos.x);
int ypos = prefs.getInt(PREFIX + "locy", pos.y);
pos.setLocation(xpos, ypos);
frame.setLocation(pos.x, pos.y);
}
private void saveLocation() {
prefs.putInt(PREFIX + "locx", pos.x);
prefs.putInt(PREFIX + "locy", pos.y);
prefs.putInt(PREFIX + "dimw", dim.width);
prefs.putInt(PREFIX + "dimh", dim.height);
try {
prefs.flush();
} catch (BackingStoreException e) {
e.printStackTrace();
}
}
View in GitHub: Java, KotlinDescription
上記のサンプルでは、対象フレームが最大化、最小化された状態で終了した場合、その前の位置サイズを記憶するよう設定しています。
Reference
- Preferences (Java Platform SE 8)
- Preferences APIの概要
- メモ:
Java 6
のPreferences API の概要にある「Java
コレクションAPI
の設計に関するFAQ
」は「Preferences API
の設計に関するFAQ
」の間違い Java 8
の「Preferences API
の概要」で修正済み
- メモ:
- PersistenceServiceを使ってJFrameの位置・サイズを記憶