GeneralPathなどで星型図形を作成する
Total: 15077
, Today: 1
, Yesterday: 4
Posted by aterai at
Last-modified:
Summary
GeneralPath
などを使って星型の図形をパネルに描画したり、アイコンを作成します。
Screenshot
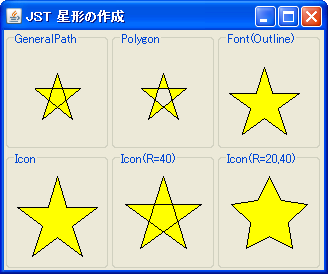
Advertisement
Source Code Examples
class StarPanel1 extends JPanel {
@Override protected void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D) g.create();
int w = getWidth();
int h = getHeight();
GeneralPath p = new GeneralPath();
p.moveTo(-w / 4f, -h / 12f);
p.lineTo(w / 4f, -h / 12f);
p.lineTo(-w / 6f, h / 4f);
p.lineTo(0f, -h / 4f);
p.lineTo(w / 6f, h / 4f);
p.closePath();
g2.translate(w / 2, h / 2);
g2.setColor(Color.YELLOW);
g2.fill(p);
g2.setColor(Color.BLACK);
g2.draw(p);
g2.dispose();
}
}
class StarIcon2 implements Icon {
private static final int R1 = 20;
private static final int R2 = 40;
private static final int VC = 5; // 16;
private final AffineTransform at;
private final Shape star;
public StarIcon2() {
double agl = 0d;
double add = 2 * Math.PI / (VC * 2);
Path2D p = new Path2D.Double();
p.moveTo(R2 * 1, R2 * 0);
for (int i = 0; i < VC * 2 - 1; i++) {
agl += add;
if (i % 2 == 0) {
p.lineTo(R1 * Math.cos(agl), R1 * Math.sin(agl));
} else {
p.lineTo(R2 * Math.cos(agl), R2 * Math.sin(agl));
}
}
p.closePath();
at = AffineTransform.getRotateInstance(-Math.PI / 2, R2, 0);
star = new Path2D.Double(p, at);
}
@Override public int getIconWidth() {
return 2 * R2;
}
@Override public int getIconHeight() {
return 2 * R2;
}
@Override public void paintIcon(Component c, Graphics g, int x, int y) {
Graphics2D g2 = (Graphics2D) g.create();
g2.translate(x, y);
g2.setPaint(Color.YELLOW);
g2.fill(star);
g2.setPaint(Color.BLACK);
g2.draw(star);
g2.dispose();
}
}
View in GitHub: Java, KotlinDescription
- 上段、左
GeneralPath
(=Path2D.Float
)を使用して星型図形を作成%JAVA_HOME%/demo/jfc/Java2D/src/java2d/demos/Lines/Joins.java
を参考
- 上段、中
Polygon
を使用して星型図形を作成
- 上段、右
Font
から星型文字★(U+2605
)のアウトラインを取得して描画
- 下段、左
10
個の頂点を予め計算してGeneralPath
で星型を作成- ついにベールを脱いだJavaFX:第9回 アニメーションを用いてより魅力的に[応用編]|gihyo.jp … 技術評論社 を参考
- 下段、中
Icon#paintIcon(...)
メソッドをオーバーライドしてPath2D.Double
で星型図形を描画- 外周の半径:
40px
- 下段、右
Icon#paintIcon(...)
メソッドをオーバーライドしてPath2D.Double
で星型図形を描画- 外周の半径:
40px
- 内周の半径:
20px
Reference
- GeneralPath (Java Platform SE 8)
- Polygon (Java Platform SE 8)
- Path2D (Java Platform SE 8)
%JAVA_HOME%/demo/jfc/Java2D/src/java2d/demos/Lines/Joins.java
- ついにベールを脱いだJavaFX:第9回 アニメーションを用いてより魅力的に[応用編]|gihyo.jp … 技術評論社
- プログラマメモ2: 扇形っぽいのを描く
- PathIteratorからSVGを生成